JavaScript proposal array grouping
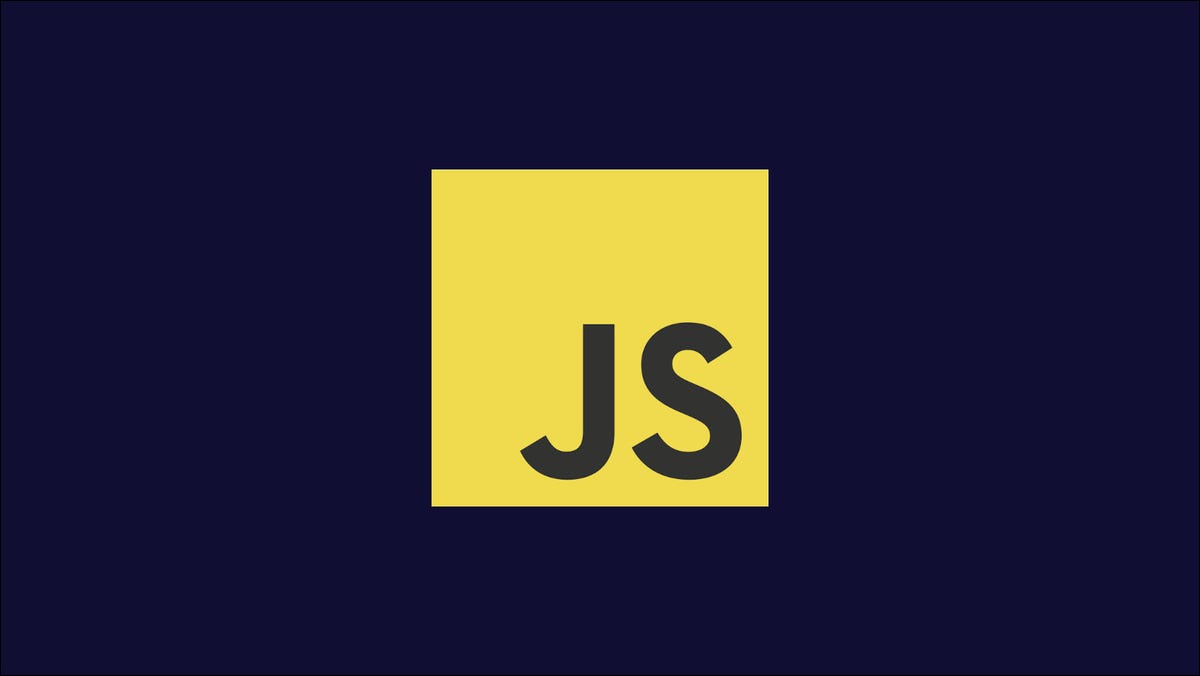
At the moment of this writing two brand-new array methods named groupBy
and groupByToMap
are getting closer to official release since they are in stage 3.
For years, we used to pull Lodash groupBy or even write our own custom utility methods to group array items, so now it seems JavaScript is going to provide this out of the box.
Let's see an example for groupBy
:
We can also use groupByToMap
to end up with a Map:
Here is a Codesandbox you can use to play with these two pretty helpful methods straight ahead.
Notice the imported modules core-js/actual/array/group-by
and core-js/actual/array/group-by-to-map
I had to pull from core-js to make these two work. Cheers!!
Did you like this one?
I hope you really did...
Newsletter
Get notified about latest posts and updates once a week!!
You liked it?